ROS Python Programming for Robotics
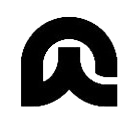

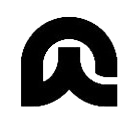
ROS Python Programming for Robotics: Your Gateway to Intelligent Machines
Robotics is booming, and at the heart of many successful robotic systems lies the Robot Operating System (ROS). A flexible framework, ROS allows developers to build complex robotic applications by breaking them down into smaller, manageable nodes that communicate with each other. And while ROS supports various programming languages, Python has emerged as a favorite due to its ease of use, extensive libraries, and rapid prototyping capabilities. This post will delve into the world of ROS Python programming, highlighting its advantages and guiding you through the essentials.
Why Python for ROS?
Several factors contribute to Python's popularity in the ROS ecosystem:
-
Ease of Use and Readability: Python's syntax is remarkably intuitive and readable, making it easier for beginners to learn and experienced developers to maintain large codebases. This is crucial in robotics, where projects often involve multiple contributors and require extensive debugging.
-
Rich Ecosystem of Libraries: Python boasts a vast collection of libraries for numerical computation (NumPy), data manipulation (Pandas), visualization (Matplotlib), and machine learning (Scikit-learn, TensorFlow, PyTorch). These libraries seamlessly integrate with ROS, providing powerful tools for sensor processing, control algorithms, and AI integration.
-
Rapid Prototyping: Python's dynamic typing and interpreted nature allow for quick development cycles. You can test your code incrementally and iterate quickly, crucial for the experimental nature of robotics research and development.
-
Large Community Support: The ROS community is vibrant and supportive, with numerous online resources, tutorials, and forums dedicated to Python-based ROS development. This makes it easier to find solutions to problems and learn from experienced developers.
Getting Started with ROS Python
Before diving into code, you'll need to install ROS (the specific version depends on your operating system; consult the official ROS documentation). Once ROS is installed, you'll need to install the rospy
package, the primary Python library for interacting with ROS. This can typically be done using your distribution's package manager (e.g., apt-get install ros-<distro>-rospy
for Debian/Ubuntu).
Basic ROS Concepts in Python
Understanding fundamental ROS concepts is essential:
-
Nodes: Independent processes that perform specific tasks, like reading sensor data, controlling actuators, or processing images. In Python, these are created using the
rospy.init_node()
function. -
Topics: Channels for publishing and subscribing to data. Data is transmitted as messages, defined using ROS message definition files (.msg). In Python, you publish using
rospy.Publisher
and subscribe usingrospy.Subscriber
. -
Services: Request-response mechanisms for more complex interactions between nodes. Services are defined using ROS service definition files (.srv). In Python, you create service clients and servers using
rospy.ServiceProxy
androspy.Service
. -
Messages: Data structures used to communicate information between nodes. These are defined in .msg files and automatically generated into Python classes.
Example: A Simple Publisher and Subscriber
Let's illustrate a basic ROS Python program: a publisher that publishes a number and a subscriber that prints the received number.
# Publisher
import rospy
from std_msgs.msg import Int32
rospy.init_node('number_publisher')
pub = rospy.Publisher('number', Int32, queue_size=10)
rate = rospy.Rate(1) # 1 Hz
while not rospy.is_shutdown():
num = 10
rospy.loginfo(f"Publishing: {num}")
pub.publish(num)
rate.sleep()
# Subscriber
import rospy
from std_msgs.msg import Int32
def callback(data):
rospy.loginfo(rospy.get_caller_id() + "I heard %s", data.data)
rospy.init_node('number_subscriber')
rospy.Subscriber("number", Int32, callback)
rospy.spin()
This code demonstrates the creation of a publisher node (number_publisher
) that sends integer values on the 'number' topic and a subscriber node (number_subscriber
) that receives and prints those values. Remember to run these two nodes in separate terminals.
Advanced ROS Python Techniques
Beyond basic publishing and subscribing, ROS Python allows for advanced functionalities:
-
Actions: For complex tasks requiring feedback and preemption. Actions provide a more robust way to control robotic systems compared to simple topics.
-
Parameter Server: A centralized storage for configuration parameters, accessible by all nodes. This allows for easy modification of robot behavior without recompiling code.
-
TF Transformations: For managing coordinate frames and transformations between different parts of the robot. This is crucial for integrating sensors and actuators that operate in different coordinate systems.
-
RViz: A 3D visualization tool that allows you to see your robot's state, sensor data, and simulated environment. Python scripts can interact with RViz to display custom visualizations.
Conclusion
ROS Python programming provides a powerful and efficient way to develop robotic applications. Its ease of use, extensive libraries, and strong community support make it an ideal choice for both beginners and experienced developers. While this introduction covers the fundamentals, there's much more to explore. By mastering these core concepts and leveraging the vast resources available, you can build sophisticated robotic systems and push the boundaries of what's possible in the field. So, start coding, experiment, and unlock the potential of ROS Python in your robotic endeavors!