ROS Packages Code Organization
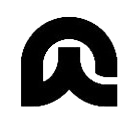

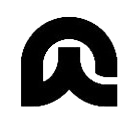
ROS Packages: A Deep Dive into Code Organization
ROS (Robot Operating System) is a powerful framework for robotics development, but its effectiveness hinges on well-organized code. A messy, disorganized package can quickly become a nightmare to maintain, debug, and collaborate on. This blog post will delve into best practices for organizing your ROS packages, ensuring your projects remain manageable and scalable.
The Foundation: ROS Package Structure
Before we dive into organization strategies, let's review the fundamental structure of a ROS package. At its core, a ROS package is a directory containing various files and subdirectories. The most crucial components include:
-
package.xml
: This XML file is the heart of your package. It declares the package's name, version, dependencies (other packages your code relies on), and authors. This is essential for ROS to understand and manage your package. -
CMakeLists.txt
: This file is crucial for building your package. It describes the source code, executables, libraries, and other resources to be compiled. UnderstandingCMakeLists.txt
is fundamental to ROS development. -
src/
: This directory holds the actual source code of your package. This is where your C++, Python, or other language files reside. Organizing this directory effectively is the focus of this post. -
include/
: (Optional but recommended) This directory contains header files (.h files in C++) that declare classes, functions, and other elements used by your source code.
Other common components include:
launch/
: Contains launch files (.launch) that describe how to start and configure your nodes.config/
: Holds configuration files (e.g., YAML files) used to parameterize your nodes.test/
: Contains unit tests for your code.data/
: Contains any necessary data files like maps, models, or other resources.
Best Practices for Code Organization Within src/
The src/
directory often becomes the most complex part of a ROS package. Here's how to keep it manageable:
1. Modular Design: Break down your code into smaller, self-contained modules. Each module should have a specific purpose and ideally reside in its own subdirectory within src/
. This promotes code reusability and simplifies maintenance. For example, a robotics project might have separate modules for:
motion_planning/
: Contains algorithms for path planning and motion control.perception/
: Handles sensor data processing and object recognition.control/
: Implements control algorithms for actuators.visualization/
: Provides tools for visualizing robot state and sensor data.
2. Consistent Naming Conventions: Adopt clear and consistent naming conventions for files, classes, and functions. This greatly improves code readability and understanding. Using a consistent style guide (e.g., Google C++ Style Guide) is highly recommended.
3. Meaningful Directory Names: Choose descriptive and self-explanatory names for your subdirectories. Avoid generic names like "util" or "helper" unless the purpose is truly general-purpose.
4. Utilize Namespaces: In C++, using namespaces prevents naming collisions between different parts of your code and external libraries. This is crucial as your project grows in complexity.
5. Documentation: Thorough documentation is vital. Use comments to explain the purpose and functionality of your code. Consider using a documentation generator like Doxygen to create comprehensive documentation from your code's comments.
6. Version Control: Use a version control system like Git to track changes to your code. This allows you to revert to earlier versions if necessary, collaborate with others effectively, and maintain a history of your project's development.
7. Unit Testing: Write unit tests to verify the correctness of your code. Include a test/
directory within your package, and use a testing framework like Google Test to write and run your tests.
8. Separation of Concerns: Separate concerns like algorithms, data structures, and user interfaces into different files and modules. This improves code organization and reduces dependencies between different parts of your code.
Example: Organizing a Simple Navigation Package
Let's imagine a ROS package for robot navigation. A well-organized structure might look like this:
navigation_package/
├── CMakeLists.txt
├── package.xml
├── src/
│ ├── navigation.cpp
│ ├── path_planning/
│ │ ├── astar.cpp
│ │ ├── astar.h
│ │ └── rrt.cpp
│ │ └── rrt.h
│ ├── control/
│ │ ├── pid_controller.cpp
│ │ └── pid_controller.h
│ └── utils/
│ ├── geometry.cpp
│ └── geometry.h
├── include/
│ ├── navigation.h
│ ├── path_planning/
│ │ ├── astar.h
│ │ └── rrt.h
│ └── control/
│ └── pid_controller.h
├── launch/
│ └── navigation.launch
├── config/
│ └── navigation_params.yaml
└── test/
└── test_navigation.cpp
This structure clearly separates path planning, control, and utility functions, making the code easier to understand, maintain, and extend.
Conclusion
Careful code organization is not merely a matter of aesthetics; it’s crucial for the long-term success of any ROS project. By following these best practices, you can build maintainable, scalable, and collaborative ROS packages that simplify development and accelerate your robotics projects. Remember, clean code is happy code, and happy code leads to happy robots!