ROS Messages Custom Data Structures
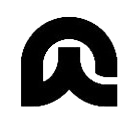

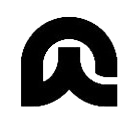
ROS Messages: Crafting Your Custom Data Structures
ROS (Robot Operating System) relies heavily on message passing for communication between nodes. While ROS provides a plethora of standard message types (like Int32
, Float64
, String
), the real power unlocks when you create your own custom message structures to represent the unique data flowing through your robot's systems. This blog post will guide you through the process of designing and implementing custom ROS messages, empowering you to tailor your communication to specific robotic applications.
Why Custom Messages Matter
Imagine building a robot that uses a laser scanner, a camera, and an IMU (Inertial Measurement Unit). Each sensor produces data in a specific format. Using only standard ROS message types would force you to split this data into numerous messages, making integration complex and inefficient. A custom message, however, can neatly encapsulate all the relevant sensor data into a single, well-organized structure. This simplifies your code, improves readability, and significantly boosts performance by reducing the overhead of individual message passing.
Defining Your Message Structure
The first step is meticulously planning your message's contents. Consider the following:
-
Data Types: Choose the most appropriate ROS data types for each piece of information. This might include built-in types like
int8
,float32
,string
, or more complex types like arrays, booleans, or even other custom messages (nested messages). -
Data Organization: Structure your data logically. Group related data together to improve readability and maintainability. For example, if your message contains sensor data, you might group the sensor ID, timestamp, and sensor readings together.
-
Clarity and Consistency: Use descriptive names for your message fields. Maintain consistency in naming conventions (e.g., camelCase or snake_case) throughout your message.
Creating the Message Definition File (.msg)
ROS messages are defined using a simple text-based language in files with the .msg
extension. These files describe the structure of your message, specifying the name and data type of each field.
Let's create a custom message called SensorData
that combines data from a laser scanner and an IMU:
# SensorData.msg
# Laser Scanner Data
float32[] laser_scan
# IMU Data
float32 imu_roll
float32 imu_pitch
float32 imu_yaw
float32 imu_acceleration_x
float32 imu_acceleration_y
float32 imu_acceleration_z
# Timestamp
time timestamp
This .msg
file defines our SensorData
message. Notice how we've grouped the laser scan data and IMU data separately and included a timestamp for accurate time synchronization.
Building the Message
After creating your .msg
file, you need to build it using the catkin_make
command (or the equivalent for your build system). This generates the necessary C++ and Python code for using your custom message. The location of the generated files depends on your ROS installation, usually within the devel
or build
directories of your workspace.
Using Your Custom Message in Your ROS Nodes
Now that your message is built, you can use it in your ROS nodes. Here's an example of a publisher node in Python:
#!/usr/bin/env python
import rospy
from your_package.msg import SensorData # Replace your_package
def talker():
pub = rospy.Publisher('sensor_data', SensorData, queue_size=10)
rospy.init_node('sensor_data_publisher', anonymous=True)
rate = rospy.Rate(10) # 10hz
while not rospy.is_shutdown():
msg = SensorData()
# Populate the message with sensor data
msg.laser_scan = [1.0, 2.0, 3.0] # Example laser scan data
msg.imu_roll = 0.1
# ... populate other fields ...
msg.timestamp = rospy.Time.now()
pub.publish(msg)
rate.sleep()
if __name__ == '__main__':
try:
talker()
except rospy.ROSInterruptException:
pass
This code snippet shows how to create a publisher node that publishes messages of type SensorData
. Remember to replace your_package
with the actual name of your ROS package. You would similarly use a subscriber node to receive and process these messages.
Advanced Techniques
-
Nested Messages: You can embed custom messages within other custom messages to create hierarchical data structures.
-
Arrays and Dynamic Arrays: Use arrays to represent collections of data. Dynamic arrays allow the size of the array to change at runtime.
-
Header Information: Including a
std_msgs/Header
as the first field of your message is a common practice. This header typically contains a timestamp and frame ID, useful for synchronizing and spatially referencing data.
Conclusion
Creating custom ROS messages is a crucial skill for any serious ROS developer. It allows you to represent your robot's data in a clean, organized, and efficient manner, leading to more robust, maintainable, and scalable robotic systems. By carefully designing your message structures and adhering to best practices, you can significantly improve the clarity and performance of your ROS applications. Remember to meticulously plan your message structure, choose appropriate data types, and use descriptive names to ensure the clarity and maintainability of your code. Happy robotizing!