ROS C++ Programming for Robotics
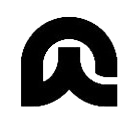

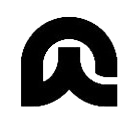
ROS C++ Programming for Robotics: A Deep Dive
Robotics is a fascinating field, constantly pushing the boundaries of what's possible. At the heart of many advanced robotic systems lies ROS (Robot Operating System), a powerful and flexible framework. While ROS supports various programming languages, C++ remains a dominant choice due to its performance, control, and extensive library support. This blog post explores the intricacies of ROS C++ programming, guiding you through its key concepts and empowering you to build sophisticated robotic applications.
Why C++ for ROS?
Choosing a programming language for robotics is crucial. C++ offers several compelling advantages within the ROS ecosystem:
-
Performance: C++ is a compiled language, resulting in significantly faster execution speeds compared to interpreted languages like Python. This is particularly vital for real-time robotic applications requiring precise timing and responsiveness. For tasks like low-level motor control or image processing, the performance difference can be substantial.
-
Memory Management: C++ provides fine-grained control over memory management, enabling efficient resource utilization. This is especially important in resource-constrained robotic systems where memory and processing power are limited.
-
Extensive Libraries: The ROS ecosystem boasts a wealth of C++ libraries for various robotic tasks, including navigation, perception, and manipulation. These libraries provide pre-built functionalities, accelerating development and simplifying complex implementations.
-
Community Support: A large and active community supports ROS C++ development. Abundant online resources, tutorials, and forums make troubleshooting and learning easier.
Getting Started: Setting up your ROS C++ Environment
Before diving into code, you need a properly configured ROS environment. This involves:
-
Installing ROS: The installation process varies based on your operating system (Ubuntu is commonly used). Consult the official ROS documentation for detailed instructions.
-
Setting up your workspace: ROS uses workspaces to organize your code, packages, and dependencies. You'll create a workspace and then build your ROS packages within it using
catkin
(orcolcon
for newer ROS distributions). -
Familiarizing yourself with basic ROS concepts: Understand essential concepts like nodes, topics, services, and actions. These form the building blocks of any ROS application.
Core ROS C++ Components
Let's delve into the key components you'll work with in ROS C++ programming:
-
Nodes: These are the fundamental units of computation in ROS. Each node performs a specific task, such as reading sensor data, controlling actuators, or processing images. Nodes communicate with each other through topics, services, or actions.
-
Topics: Topics are used for asynchronous communication between nodes. A node publishes data to a topic, and other nodes subscribe to that topic to receive the data. This is ideal for streaming data like sensor readings or camera images.
-
Services: Services facilitate synchronous communication. A node requests a service from another node, and the service-providing node performs a task and returns a response. This is suitable for requests that require a specific action and a response, such as setting a robot's joint angles.
-
Actions: Actions are used for complex, long-running tasks that may require feedback and preemption. They provide a robust mechanism for managing complex operations like navigation or manipulation.
Writing a Simple ROS C++ Node
Let's create a basic ROS C++ node that publishes a message to a topic:
#include <ros/ros.h>
#include <std_msgs/String.h>
int main(int argc, char **argv) {
ros::init(argc, argv, "hello_world_node");
ros::NodeHandle nh;
ros::Publisher pub = nh.advertise<std_msgs::String>("hello_topic", 10);
ros::Rate loop_rate(1); // Publish at 1Hz
while (ros::ok()) {
std_msgs::String msg;
msg.data = "Hello, ROS!";
pub.publish(msg);
ros::spinOnce();
loop_rate.sleep();
}
return 0;
}
This code snippet initializes a node, creates a publisher, and publishes a "Hello, ROS!" message to the "hello_topic" topic at a rate of 1Hz.
Advanced Topics and Libraries
Once you've grasped the basics, you can explore more advanced concepts:
-
ROS Parameter Server: Use the parameter server to store and retrieve configuration parameters for your nodes. This allows you to easily modify settings without recompiling your code.
-
ROS Services: Implement custom services to handle complex requests and responses between your nodes.
-
ROS Actions: Leverage actions for long-running, complex tasks that require feedback and cancellation capabilities.
-
TF (Transform Library): Use the TF library to manage coordinate transforms between different coordinate frames in your robot. This is crucial for integrating sensor data and controlling robot movements.
-
RViz: RViz is a powerful 3D visualization tool that allows you to visualize your robot's state, sensor data, and other information in real-time.
-
MoveIt!: MoveIt! is a powerful motion planning framework that simplifies the process of planning robot movements and avoiding collisions.
Conclusion
ROS C++ programming offers a powerful and efficient way to develop complex robotic applications. While the initial learning curve might seem steep, the performance advantages, extensive library support, and active community make it a worthwhile investment for any serious robotics developer. By understanding the fundamental concepts of nodes, topics, services, and actions, and by utilizing the wealth of available libraries and tools, you can unlock the full potential of ROS C++ to build innovative and sophisticated robotic systems. Start with the basics, experiment with example code, and gradually delve into more advanced topics – the world of robotics awaits!